The XabcdAnnotation allows a user to draw a Harmonic or Gartley or Bat pattern on a price chart. To create this annotation you have to set points at critical turning-points that will be connected by lines and polygons. Those points were limited by BasePointsCount (default value is 6).
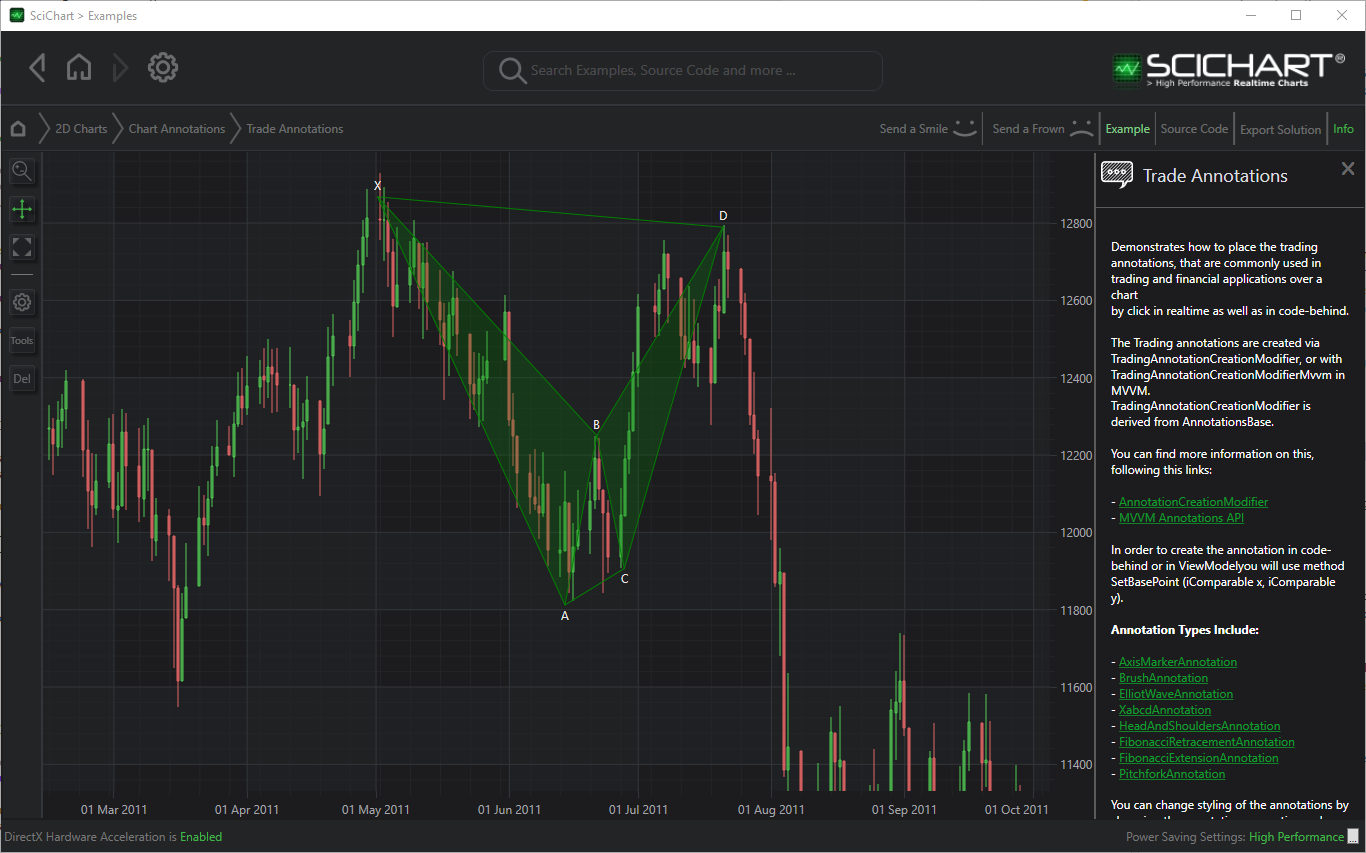
The XabcdAnnotation is a CompositeAnnotation (an annotation which consists of a number of other annotations) which consists of:
- LineAnnotations - which are connecting our points XA, AB, XB, AC, BC, CD, BD and XD
- PolygonAnnotations - that are placed inside the triangles formed by our points XAB and BCD
- Text labels - which are TextAnnotations
Additional properties specific to XabcdAnnotation include:
- Fill - defines the style for the polygon fils
Adding XabcdAnnotations via mouse click
To add an XabcdAnnotation via mouse click you have to add TradingAnnotationCreationModifier to your SciChartSurface.ChartModifier and set AnnotationType as XabcdAnnotation. Please see the article on the TradingAnnotationCreationModifier for how to use this type.
Declaring an XabcdAnnotation in XAML
To declare an XabcdAnnotation in XAML you have to declare the annotation and then declare its InitialBasePoints collection that is actually collection of ComparablePoint objects. ComparablePoint stores X and Y values that are used for placing annotation point. InitialBasePoints collection is limited by BasePointsCount value. And default value is 6.
Declaring an XabcdAnnotation in XAML |
Copy Code |
---|---|
<!-- XAxis, YAxis, RenderableSeries omitted for brevity --> <s:SciChartSurface.Annotations> <!--Declare XabcdAnnotation--> <s:XabcdAnnotation IsEditable="True"> <!-- Declare InitialBasePoints collection that is used for creating annotation from XAML. InitialBasePoints is actually observable collection of ComparablePoint-s. ComparablePoint stores X and Y point Value. --> <s:XabcdAnnotation.InitialBasePoints> <!-- Placing X point --> <s:ComparablePoint X="0" Y="0"/> <!-- Placing A point --> <s:ComparablePoint X="2" Y="4"/> <!-- Placing B point --> <s:ComparablePoint X="4" Y="0"/> <!-- Placing C point --> <s:ComparablePoint X="6" Y="5"/> <!-- Placing D point --> <s:ComparablePoint X="10" Y="-2"/> </s:XabcdAnnotation.InitialBasePoints> </s:XabcdAnnotation> </s:SciChartSurface.Annotations> |
Please Note that the XabcdAnnotation has limit of 6 base points. As you can see here, you just have to set this 6points for XabcdAnnotation : [X1=0; Y1=0], [X2=2; Y2=4], [X3=4; Y3=0], [X4=6; Y4=-4], [X5=9; Y5=0], [X6=11; Y6=-4] .
Declaring an XabcdAnnotation in Code-Behind
To add an XabcdAnnotation in code, simply use the following C# code:
Declaring the XabcdAnnotation in Code |
Copy Code |
---|---|
// Create a SciChartSurface. XAxis, YAxis omitted for brevity var sciChartSurface = new SciChartSurface(); // Create a XABCDAnnotation. var xabcd = new XabcdAnnotation(); // XABCDAnnotation should be added to //SciChartSurface.Annotation collection first, to make SetBasePoint() method //works correctly sciChart.Annotations.Add(xabcd); // Set X point of XABCDAnnotation xabcd.SetBasePoint(0, 0); // Set A point of XABCDAnnotation xabcd.SetBasePoint(2, 4); // Set B point of XABCDAnnotation xabcd.SetBasePoint(4, 0); // Set C point of XABCDAnnotation xabcd.SetBasePoint(6, -4); // Set D point of XABCDAnnotation xabcd.SetBasePoint(9, 0); |
Declaring an XabcdAnnotation in MVVM
To add an XabcdAnnotation via MVVM please follow the instructions for adding annotations with MVVM: Declaring Annotations in MVVM with the AnnotationsBinding Markup Extension
To add a XabcdAnnotation via a ViewModel you have to use SciChart markup extension called AnnotationsBinding,
The AnnotationsBinding Markup Extension |
Copy Code |
---|---|
<s:SciChartSurface Annotations="{s:AnnotationsBinding Annotations}"> </s:SciChartSurface> |
Now you need to create collection for Annotations in ViewModel. Then create and add an XabcdAnnotationViewModel to that collection of Annotations like this:
Declaring the XabcdAnnotation in a ViewModel |
Copy Code |
---|---|
public class MyViewModel : INotifyPropertyChanged { public ObservableCollection<IAnnotationViewModel> Annotations { get; } public void Foo() { // Create a XabcdAnnotation. xabcd = new XabcdAnnotationViewModel(); // XabcdAnnotation should be added to //SciChartSurface.Annotation collection first, to make SetBasePoint() method //works correctly Annotations.Add(xabcd); // Set X point of XABCDAnnotation xabcd.SetBasePoint(0, 0); // Set A point of XABCDAnnotation xabcd.SetBasePoint(2, 4); // Set B point of XABCDAnnotation xabcd.SetBasePoint(4, 0); // Set C point of XABCDAnnotation xabcd.SetBasePoint(6, -4); // Set D point of XABCDAnnotation xabcd.SetBasePoint(9, 0); } } |