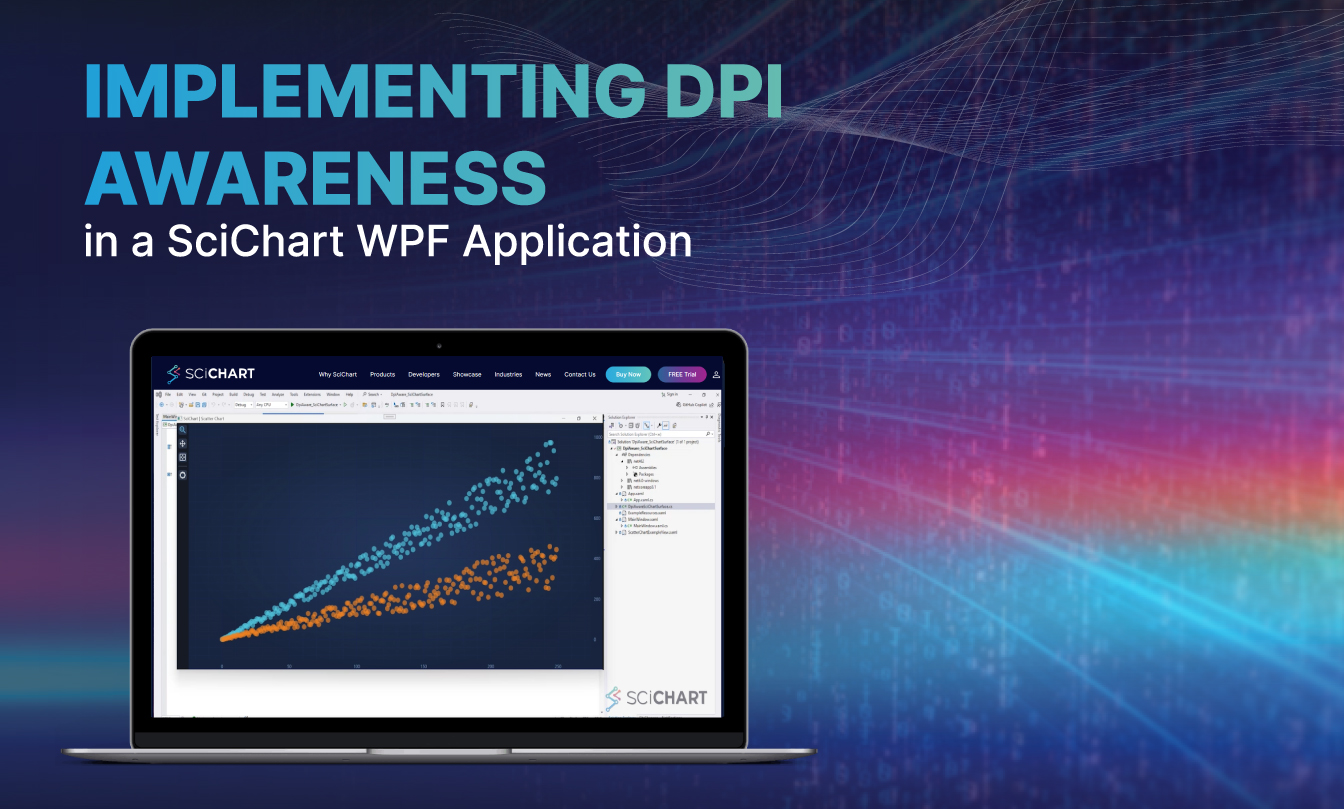
Implementing DPI Awareness in a WPF Charting Application
As modern software environments increasingly span across multiple monitors with different resolutions and pixel densities, it’s essential that WPF applications remain crisp and clear across all displays. DPI (Dots Per Inch) awareness ensures that UI elements, text, and charts do not appear blurry when moved between monitors with different DPI settings.
In this tutorial, we will walk through the process of making a SciChart WPF application DPI aware. By modifying the application manifest and applying a few adjustments to your charting controls, you can ensure your application scales correctly and maintains visual clarity across all monitors.
Step 1: Adding the DPI Awareness Manifest
To make your WPF application DPI aware, you first need to modify the application manifest file. The manifest specifies how your application should behave when moved between displays with different DPI settings.
1.1. Add a Manifest File: In Visual Studio, right-click your project, select Add, and then choose New Item. Search for Application Manifest and add it to your project.
1.2. Modify the Manifest: The following XML snippet should be added to the manifest file. It ensures Per-Monitor DPI awareness for systems running Windows 10 Anniversary Update or newer, and provides compatibility for systems running older versions of Windows.
<application xmlns="urn:schemas-microsoft-com:asm.v3">
<windowsSettings>
<!-- The combination of below two tags have the following effect: -->
<!-- 1) Per-Monitor for >= Windows 10 Anniversary Update -->
<!-- 2) System < Windows 10 Anniversary Update -->
<dpiAwareness xmlns=" http://schemas.microsoft.com/SMI/2016/WindowsSettings"> PerMonitor</dpiAwareness>
<dpiAware xmlns=" http://schemas.microsoft.com/SMI/2005/WindowsSettings">true</dpiAware>
</windowsSettings>
</application>
1.3. Build and Test: After modifying the manifest, rebuild your application. Moving the app between monitors with different DPI settings should now result in a sharp, clear interface without any blurring or distortion.
A. Before enabling DPI awareness, the chart may appear blurry on high-resolution displays.
B. After making the application DPI aware, the chart renders sharply, maintaining visual clarity across monitors.
Step 2: Overriding DPI Change Handling in SciChart
Once the manifest is in place, the next step is ensuring that your application dynamically adjusts when the DPI changes. For SciChart WPF applications, this involves overriding the OnDpiChanged method within your SciChartSurface to apply scaling transformations based on the current DPI settings.
protected override void OnDpiChanged(DpiScale oldDpi, DpiScale newDpi)
{
var scaleFactorX = newDpi.DpiScaleX;
var scaleFactorY = newDpi.DpiScaleY;
// Apply scaling transformation
LayoutTransform = new ScaleTransform(scaleFactorX, scaleFactorY);
}
This method calculates the new DPI scale factors for both the X and Y coordinates and applies these to the SciChartSurface to ensure the charts and other UI elements remain sharp and proportional.
Step 3: Ensuring Bitmap Scaling Mode
In WPF, the default bitmap scaling mode can cause blurring during DPI adjustments. By setting the BitmapScalingMode to NearestNeighbor, you ensure that the application renders images and charts without interpolation, preserving clarity even at high DPI values.
RenderOptions.SetBitmapScalingMode(chartSurface, BitmapScalingMode.NearestNeighbor);
This step ensures that all visual elements, including charts, labels, and icons, retain their sharpness, particularly when users scale their display or move the application across monitors.
Step 4: Testing DPI Awareness
With these adjustments in place, you can now test your application on different monitors with varying DPI settings. The application should remain sharp and clear regardless of the monitor it is displayed on. Specifically, check for:
- Text labels remaining sharp.
- Charts and grid lines rendering without distortion.
- Overall UI consistency as you move the window between monitors with different DPIs.
Making DPI Awareness Standard in SciChart WPF Applications
By implementing DPI awareness in your SciChart WPF application, you ensure that it performs optimally across different display setups. Whether the user is working on a 1080p monitor or a 4K display, the charts, UI, and text will retain their clarity and sharpness, providing a consistent and professional user experience.
Watch the Full Video Tutorial
For a detailed walkthrough on enabling DPI awareness in SciChart WPF, watch our full tutorial here:
Find Out More
Visit our documentation and GitHub repository for more examples on how to build high-performance, DPI-aware applications with SciChart WPF DpiAware_SciChartSurface examples in sandbox.
Recent Blogs